In many situations we store important configuration values in XML/Config files. Especially when we use Cloud Systems, it is not safe to store important data in plain text.
Below is one simple way that I used to encrypt & decrypt this data in Config files.
There are several encryption & decryption algorithms supported by .NET. Refer this for details.
There are a lot of things in the field of encryption. This post will show you all you need for encrypting a string to cipher text and decrypt it back to regular string. I decided to use AES with 256 bit encryption. A similar example is found here.
Below are the simple steps.
1. Identify the Block Size and Key Size for the Encryption algorithm you chose (this number is in bits not bytes). You can assign a different value to these properties. To see the minimum and maximum block sizes supported by the algorithm, use LegalKeySizes and LegalBlockSizes properties.
2. Define Encryption Key and IV (Initialization Vector) for the algorithm. These are strings which are used to encrypt and decrypt plain text.
3. Encryption Key should be equal to the Key size for the algorithm. Since, my key size is 256 bits; I need to create a key which should give 256 bits upon encoding it.
4. IV should be equal to the Block size for the algorithm. Since, my block size is 128 bits; I need to create an IV which should give 128 bits upon encoding it.
5. Once you have the key and IV, use any of the encoding methods and convert the key and IV into a byte[]. Resulting byte[] should have a length of 256 for encryption key and 128 for IV.
6. You can then use this key and IV to encrypt the plain text to a cipher value and vice versa.
7. Once encrypted, the result will be again in byte[] which may contain non printable characters. Hence use base64 encoding on the resultant cipher bits to get a printable cipher string.
8. Below is the sample code. In practice you should use a very complex key and IV. Below is just for understanding. Your encrypted value is safe as long as the key and IV are safe.
In the above, Implementation of the method EncryptStringToBytes_Aes can be found in MSDN which uses CryptoStream Class.
9. To decrypt, use the same approach.
Implementation of the method DecryptStringFromBytes_Aes can be found in MSDN which uses CryptoStream Class.
10. This method will convert a simple text “Hello” into base64 encoded cipher text “EygxuhAY/Evm9wPVv2UrlA==”.
Summary: Encryption alogrithm generates cipher bits. Resultant cipher bits are converted to base64 string so that we can store it easily in a config file. Something like below.
To get the original string, convert the base64 encoded cipher to cipher bit array and call decryption method.
Key & IV should be saved in a secure place like SSO Database and read from it to perform decryption of values in config files.
HTH.
– SHiv
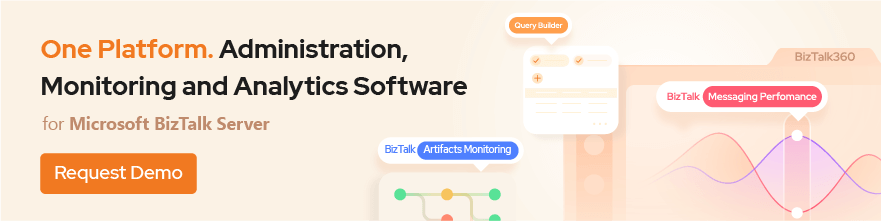